1.Python 中的if...else
语句是什么?
仅当满足特定条件时,我们才想执行代码时才需要决策。
if…elif…else
语句在 Python 中用于决策。
Python if
语句语法
if test expression: statement(s)
在此,程序将求值test expression并仅在测试表达式为True时才执行语句。
如果测试表达式为False,则不执行该语句。
在 Python 中,if语句的主体由缩进指示。 主体以缩进开始,第一条未缩进的线标记结束。
Python 将非零值解释为True。None和0解释为False。
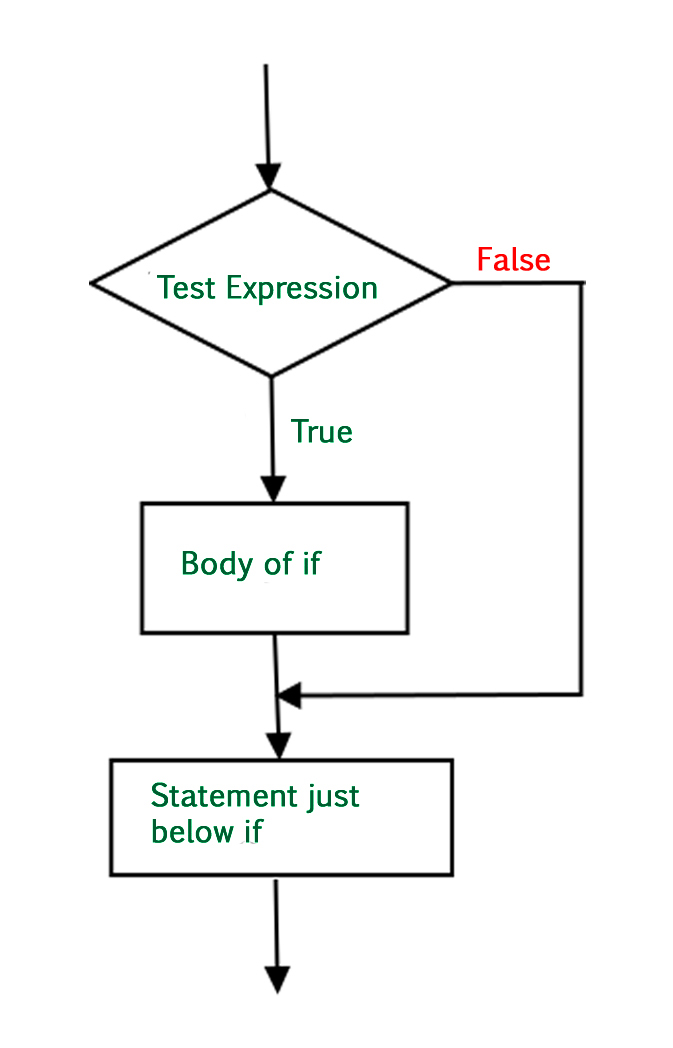
例1.1 Python if语句
# If the number is positive, we print an appropriate message num = 3 if num > 0: print(num, "is a positive number.") print("This is always printed.") num = -1 if num > 0: print(num, "is a positive number.") print("This is also always printed.")
结果
在上面的示例中,num > 0是测试表达式。
仅当if的主体的值为True时,才执行它。
当变量num等于 3 时,测试表达式为true,并且执行if主体内的语句。
如果变量num等于 -1,则测试表达式为false,并且跳过if主体内部的语句。
print()语句位于if块之外(未缩进)。 因此,无论测试表达式如何,都将执行它。
2.Python if...else
语句
if...else
的语法
if test expression: Body of if else: Body of else
if..else语句求值test expression并仅在测试条件为True时才执行if的主体。
如果条件为False,则执行else的主体。 缩进用于分隔块。
# Program checks if the number is positive or negative # And displays an appropriate message num = 3 # Try these two variations as well. # num = -5 # num = 0 if num >= 0: print("Positive or Zero") else: print("Negative number")
结果
1 |
Positive or Zero |
在上面的示例中,当num
等于 3 时,测试表达式为true
,并且执行if
的主体,其他的body
被跳过。
如果num
等于 -5,则测试表达式为false
,并且执行else
的主体,并且跳过if
的主体。
如果num
等于 0,则测试表达式为true
,并且执行if
的主体,而跳过其他的body
。
3.Python if...elif...else
语句
if...elif...else
的语法
if test expression: Body of if elif test expression: Body of elif else: Body of else
elif是if的缩写。 它允许我们检查多个表达式。
如果if的条件为False,它将检查下一个elif块的条件,依此类推。
如果所有条件均为False,则执行else的主体。
根据条件,仅执行几个if…elif…else块中的一个块。
if块只能有一个else块。 但是它可以具有多个elif块。
if…elif…else的流程图
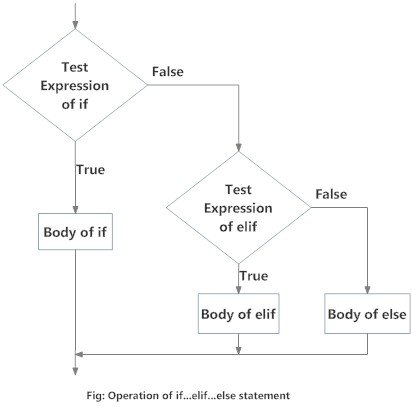
例3.1 if…elif…else的示例
'''In this program, we check if the number is positive or negative or zero and display an appropriate message''' num = 3.4 # Try these two variations as well: # num = 0 # num = -4.5 if num > 0: print("Positive number") elif num == 0: print("Zero") else: print("Negative number")
结果
1 |
Positive number |
当变量num
为正时,将打印正数。
如果num
等于 0,则打印零。
如果number
为负,则打印负数。
例3.2
# Example file for working with conditional statement # def main(): x, y = 8, 8 if (x < y): st = "x is less than y" elif (x == y): st = "x is same as y" else: st = "x is greater than y" print(st) if __name__ == "__main__": main()
结果:
1 |
x is same as y |
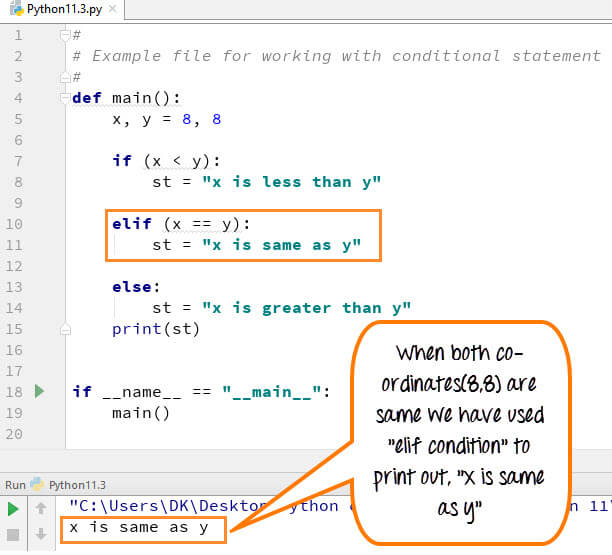
- 代码行 5:我们定义了两个变量 x, y = 8, 8。
- 代码第 7 行:if 语句检查条件 x
代码第 10 行:程序控制流程进入 elseif 条件。它检查 x==y 是否为真。 - 代码第 11 行:变量 st 设置为“x 与 y 相同”。
- 代码第 15 行:程序控制流退出 if 语句(它不会到达 else 语句)。并打印变量 st。输出是“x is same as y”,这是正确的。
4. Python 嵌套if
语句
我们可以在另一个if...elif...else
语句中包含一个if...elif...else
语句。 这在计算机编程中称为嵌套。
这些语句中的任何数目都可以彼此嵌套。 缩进是弄清楚嵌套级别的唯一方法。 它们可能会造成混淆,因此除非有必要,否则必须避免使用它们。
例4.1 Python 嵌套if
示例
'''In this program, we input a number check if the number is positive or negative or zero and display an appropriate message This time we use nested if statement''' num = float(input("Enter a number: ")) if num >= 0: if num == 0: print("Zero") else: print("Positive number") else: print("Negative number")
输入三个数的结果
例4.2.打印出不同国家的不同运输成本
total = 100 #country = "US" country = "AU" if country == "US": if total <= 50: print("Shipping Cost is $50") elif total <= 100: print("Shipping Cost is $25") elif total <= 150: print("Shipping Costs $5") else: print("FREE") if country == "AU": if total <= 50: print("Shipping Cost is $100") else: print("FREE")
结果
1 |
Shipping Cost is $25 |
5.如何用最少的代码执行条件语句
在这一步中,我们将看到如何压缩条件语句。我们可以将它们与单个代码一起使用,而不是分别为每个条件执行代码。
语法
1 |
A If B else C |
例5.1
def main(): x, y = 10, 8 st = "x is less than y" if (x < y) else "x is greater than or equal to y" print(st) if __name__ == "__main__": main()
结果
1 |
x is greater than or equal to y |
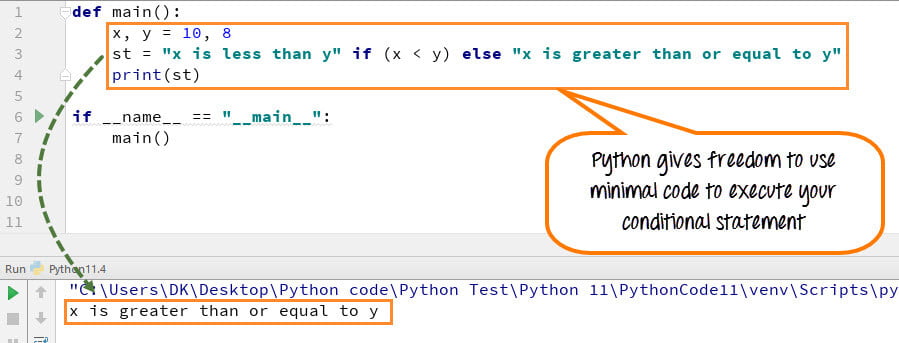
- 代码行 2:我们定义了两个变量 x, y = 10, 8
- 代码行 3:变量 st 设置为“x 小于 y”,如果 x<y,否则设置为“x 大于或等于 y”。在这个 x>y 变量中,st 被设置为“x 大于或等于 y”。
- 代码行 4:打印 st 的值并给出正确的输出
- Python 无需为条件语句编写长代码,而是让您可以自由地以简洁明了的方式编写代码。