Python 有很多可用的函数。这些函数称为内置函数。在此参考页面中,您将找到 Python 中的所有内置函数。
Python abs()
取绝对值
a = 12
b = -4
c = 3+4j
d = 7.90
print(abs(a))
print(abs(b))
print(abs(c))
print(abs(d))
结果
12
4
5.0
7.9
Python all()
循环参数,如果每个元素都为真的情况下,那么all的返回值为True:
假: 0, None, "", [],(), {}
ret = all([True, True])
ret1 = all([True, False])
result:
True
False
Python any()
只要有一个为True,则全部为True
ret = any(None, “”, [])
ret1 = any(None, “”, 1)
result:
False
True
Python ascii()
回去对象的类中,找到__repr__方法,找到该方法之后获取返回值
class Foo:
def __repr__(self):
return “hello”
obj = Foo()
ret = ascii(obj )
自动去对象(类)中找到 __repr__方法获取其返回值
Python bin()
把十进制整数转换为二进制
二进制
ret = bin(11)
result:
0b1011
Python bool()
把一个值转换为布林类型
判断真假, True:真 , False:假, 把一个对象转换成bool值
ret = bool(None)
ret = bool(1)
result:
False
True
Python bytearray()
returns array of given byte size
字节列表
列表元素是字节,
Python bytes()
returns immutable bytes object
字节
bytes(“xxx”, encoding=”utf-8″)
Python callable()
Checks if the Object is Callable
判断对象是否可被调用
class Foo: #定义类
pass
foo = Foo() # 生成Foo类实例
print(callable(foo)) # 调用实例
ret = callable(Foo) # 判断Foo类是否可被调用
print(ret)
result:
False
True
Python chr()
Returns a Character (a string) from an Integer
给数字找到对应的字符
ret = chr(65)
result:
A
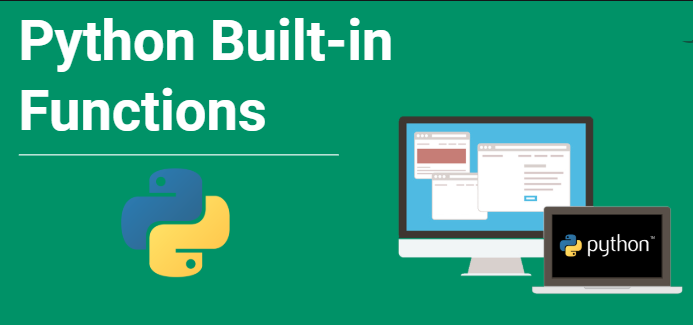
Python classmethod()
returns class method for given function
修饰符
修饰符对应的函数不需要实例化,不需要self参数,但第一个参数需要时表示自身类的cls参数,可以来调用类的属性,类的方法,实例化对象等。
class A(object):
bar = 1
def func(self):
print(“func”)
@classmethod
def func2(cls):
print(“func2”)
print(cls.bar)
cls().func() # 调用func方法
A.func2() # 不需要实例化
result:
func2
1
func
Python compile()
Returns a Python code object
函数讲一个字符串编译为字节代码
compile(source, filename, mode[, flags[, dont_inherit]])
参数:
source 字符串或者AST(Abstract Syntax Trees对象)
filename 代码文件名称,如果不是从文件读取代码则传递一些可辨认的值
mode 指定编译代码的种类,可指定: exec, eval, single
flags 变量作用域,局部命名空间,如果被提供,可以是任何映射对象。
flags和dont_inherit是用来控制编译源码时的标志
str_for = “for i in range(1,10): print(i)”
c = compile(str_for, ”, ‘exec’) # 编译为字节代码对象
print(c)
print(exec(c))
result:
1
2
3
4
5
6
7
8
9
myCode = 'a = 7\nb=9\nresult=a*b\nprint("result =",result)'
codeObject = compile(myCode, 'resultstring', 'exec')
exec(codeObject)
结果
result = 63
Python complex()
Creates a Complex Number
函数用于创建一个值为 real + image * j 的复数或者转化一个字符串或数为复数,如果第一个参数为字符串,则不需要指定第二个参数。
语法:
class complex([real ,[ image]])
参数说明:
real int, long, float或字符串
image int,long,float
ret1 = complex(1,2)
print(ret1)
ret2 = complex(1)
print(ret2)
ret3 = complex(“1”)
print(ret3)
ret4 = complex(“1+2j”)
print(ret4)
result:返回一个复数
(1+2j)
(1+0j)
(1+0j)
(1+2j)
Python delattr()
Deletes Attribute From the Object
Python dict()
Creates a Dictionary
Python dir()
Tries to Return Attributes of Object
Python divmod()
Returns a Tuple of Quotient and Remainder
Python enumerate()
Returns an Enumerate Object
Python eval()
Runs Python Code Within Program
Python exec()
Executes Dynamically Created Program
Python filter()
constructs iterator from elements which are true
Python float()
returns floating point number from number, string
Python format()
returns formatted representation of a value
Python frozenset()
returns immutable frozenset object
Python getattr()
returns value of named attribute of an object
Python globals()
returns dictionary of current global symbol table
Python hasattr()
returns whether object has named attribute
Python hash()
returns hash value of an object
Python help()
Invokes the built-in Help System
Python hex()
把整数转换为十六进制
ret = hex(14)
result:
0xe 0x:表示16进制 e: 表示14
Python id()
Returns Identify of an Object
Python input()
reads and returns a line of string
Python int()
将数字文字和字符串转换为整型
十进制
ret = int(10)
result:
10
Python isinstance()
Checks if a Object is an Instance of Class
Python issubclass()
Checks if a Class is Subclass of another Class
Python iter()
returns an iterator
Python len()
Returns Length of an Object
Python list()
creates a list in Python
Python locals()
Returns dictionary of a current local symbol table
Python map()
Applies Function and Returns a List
Python max()
returns the largest item
Python memoryview()
returns memory view of an argument
Python min()
returns the smallest value
Python next()
Retrieves next item from the iterator
Python oct()
八进制
ret = oct(14)
result:
0o16
Python open()
Returns a file object
Python ord()
returns an integer of the Unicode character
Python pow()
returns the power of a number
Python print()
Prints the Given Object
Python property()
returns the property attribute
Python range()
return sequence of integers between start and stop
Python repr()
returns a printable representation of the object
Python reversed()
returns the reversed iterator of a sequence
Python round()
rounds a number to specified decimals
Python set()
constructs and returns a set
Python setattr()
sets the value of an attribute of an object
Python slice()
returns a slice object
Python sorted()
returns a sorted list from the given iterable
Python staticmethod()
transforms a method into a static method
Python str()
returns the string version of the object
Python sum()
Adds items of an Iterable
Python super()
Returns a proxy object of the base class
Python tuple()
Returns a tuple
Python type()
Returns the type of the object
Python vars()
Returns the __dict__ attribute
Python zip()
Returns an iterator of tuples
Python __import__()
Function called by the import statement