在本教程中,你将学习如何使用 JavaScript 数组来存储一组值。
JavaScript Array 简介
数组是一种特殊类型的对象。在 JavaScript 中,数组是一个有序的值列表。每个值称为一个元素,通过索引来指定:
In JavaScript, arrays are used to store multiple values in a single variable.
在 JavaScript 中,数组用于在一个变量中存储多个值。
For example:
例如:
let colors = ['red', 'green', 'blue'];
In this example, the colors
array contains three elements: 'red'
, 'green'
, and 'blue'
.
在这个示例中,colors
数组包含三个元素:'red'
、'green'
和 'blue'
。
Each element in the array has a numeric position, known as its index, and it starts from 0.
数组中的每个元素都有一个数字位置,称为其“索引”,索引从 0 开始。
Creating an Array(创建一个数组)
There are two ways to create an array in JavaScript:
在 JavaScript 中有两种方式创建数组:
- Using an array literal(使用数组字面量)
let scores = [90, 80, 70];
- Using the
Array
constructor(使用Array
构造函数)
let scores = new Array(90, 80, 70);
In practice, it’s recommended to use array literals because they are shorter and more readable.
在实际中,推荐使用数组字面量方式,因为它更简洁、更易读。
Accessing array elements(访问数组元素)
You use square brackets []
with an index to access an array element.
你可以使用方括号 []
和索引值来访问数组元素。
For example:
例如:
let colors = ['red', 'green', 'blue'];
console.log(colors[0]); // red
console.log(colors[1]); // green
console.log(colors[2]); // blue
If you access an element with an index that is out of bounds, you’ll get undefined
:
如果你访问的索引超出数组范围,将会得到 undefined
:
console.log(colors[3]); // undefined
Modifying array elements(修改数组元素)
To change the value of an element, assign a new value using its index:
要更改数组元素的值,可以使用其索引并赋予新值:
colors[0] = 'yellow';
console.log(colors); // ['yellow', 'green', 'blue']
Array length property(数组的 length 属性)
The length
property returns the number of elements in an array:
length
属性返回数组中的元素个数:
console.log(colors.length); // 3
Iterating over elements(遍历数组元素)
You can use a for
loop to iterate over the elements of an array:
你可以使用 for
循环遍历数组中的元素:
for (let i = 0; i < colors.length; i++) {
console.log(colors[i]);
}
Array elements can be of any type(数组元素可以是任意类型)
An array can contain elements of different types:
一个数组可以包含不同类型的元素:
let random = [100, 'JavaScript', true, null];
An array can contain another array(数组可以包含另一个数组)
You can nest arrays inside arrays:
你可以在数组中嵌套另一个数组:
let scores = [
[100, 90, 80],
[95, 85, 75],
[100, 100, 100]
];
console.log(scores[0][0]); // 100
Summary(总结)
- An array is a special kind of object.
数组是一种特殊类型的对象。 - It stores values in indexed positions.
它以索引的方式存储值。 - You can create an array using the array literal syntax
[]
or theArray
constructor.
你可以使用数组字面量[]
或Array
构造函数创建数组。 - Access or modify an array element using its index.
使用索引访问或修改数组元素。 - Use the
length
property to get the number of elements in the array.
使用length
属性获取数组中的元素数量。 - Use a loop such as
for
to iterate over array elements.
使用如for
这样的循环遍历数组元素。
如需我继续翻译该网站上的更多内容或讲解数组方法(如 push
、map
、filter
等),请告诉我。
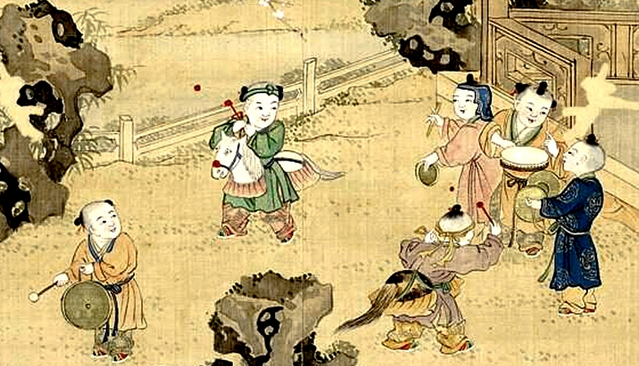
Linux, PHP, C,C++,JavaScript,verilog 老师