最常见的 Core PHP 面试问题列表,以及针对新生和有经验的求职者的详细答案和代码示例:
您想在面试中学习或测试您的 PHP 技能吗?
在本文中,我们将讨论一些最常见和最常被问到的 Core PHP 面试问题,并附有详细答案和代码示例。
对 PHP 工作的需求与日俱增。正在寻找或准备 PHP 工作的人,在面试中不得不面对一些常见的问题。因此,如果您是新人,如果您希望成为 PHP 开发人员,甚至是希望获得更高职位的经验丰富的专业人士,那么您必须阅读本文以增加您轻松快速地获得 PHP 工作的机会。
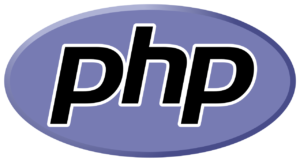
50 最常见的 PHP 面试问题
Q #1) PHP 是什么?
Answer: PHP 是一种基于脚本的 web 语言,开发者可以动态的创建网页。
PHP 的全称 是Hypertext Preprocessor(超文本预处理器)。 它通过嵌入 HTML 来创建动态内容、与数据库服务器通信、处理会话等。
Q #2) 我们为什么使用 PHP?
Answer: 使用 PHP 有几个好处。首先,它完全免费使用。因此,任何人都可以免费使用 PHP 并以最低的成本托管网站。
它支持多个数据库。最常用的数据库是 MySQL,它也可以免费使用。现在有很多 PHP 框架用于 Web 开发,例如 CodeIgniter、CakePHP、Laravel 等。
这些框架使 Web 开发任务比以前容易得多。
Q #3) PHP是强类型语言吗?
Answer: 不,PHP 是一种弱类型或松散类型的语言。
这意味着当您像其他标准编程语言 C# 或 Java 一样声明任何变量时,PHP 不需要声明变量的数据类型。当您将任何字符串值存储在变量中时,数据类型为字符串,如果您将数值存储在同一变量中,则数据类型为整数。
Sample code:
1 2 |
$var = "Hello"; //String $var = 10; //Integer |
Q #4) PHP中的可变变量是什么意思?
Answer: 当一个变量的值用作其他变量的名称时,它被称为可变变量。 $$ 用于在 PHP 中声明可变变量。
Sample code:
1 2 3 4 |
$str = "PHP"; $$str = " Programming"; //declaring variable variables echo "$str ${$str}"; //It will print "PHP programming" echo "$PHP"; //It will print "Programming" |
Q #5) echo 和 print 有什么区别 ?
Answer: echo 和 print 方法都在浏览器中打印输出,但这两种方法之间存在差异。
echo 打印输出后不返回任何值,它比 print 方法工作得更快。 print 方法比 echo 慢,因为它在打印输出后返回布尔值。
Sample code:
1 2 |
echo "PHP Developer"; $n = print "Java Developer"; |
Q #6) 如何从命令行执行 PHP 脚本?
Answer: 您必须在命令行中使用 PHP 命令来执行 PHP 脚本。如果 PHP 文件名为 test.php,则使用以下命令从命令行运行脚本。
1 |
php test.php |
Q #7) 如何在 PHP 中声明数组?
Answer: 您可以在 PHP 中声明三种类型的数组。它们是数字、关联和多维数组。
Sample code:
1 2 3 4 5 6 |
//Numeric Array $computer = array("Dell", "Lenavo", "HP"); //Associative Array $color = array("Sithi"=>"Red", "Amit"=>"Blue", "Mahek"=>"Green"); //Multidimensional Array $courses = array ( array("PHP",50), array("JQuery",15), array("AngularJS",20) ); |
Q #8) explode() 和 implode() 函数的用途是什么?
Answer: explode() 函数用于将字符串拆分为数组, implode() 函数用于通过组合数组元素来生成字符串。
Sample code:
1 2 3 4 |
$text = "I like programming"; print_r (explode(" ",$text)); $strarr = array('Pen','Pencil','Eraser'); echo implode(" ",$strarr); |
Q #9) 显示错误信息后可以使用哪个函数退出脚本?
Answer: 显示错误信息后,您可以使用 exit() 或 die() 函数退出当前脚本.
Sample code:
1 2 |
if(!fopen('t.txt','r')) exit(" Unable to open the file"); |
Sample code:
1 2 |
if(!mysqli_connect('localhost','user','password')) die(" Unable to connect with the database"); |
Q #10) PHP中使用哪个函数来检查任何变量的数据类型?
Answer: gettype() 函数用于检查任何变量的数据类型.
Sample code:
1 2 3 4 |
echo gettype(true).''; //boolean echo gettype(10).''; //integer echo gettype('Web Programming').''; //string echo gettype(null).''; //NULL |
Q #11) 如何在 PHP 中增加脚本的最大执行时间?
Answer: 您需要更改 php.ini 文件中 max_execution_time 指令的值以增加最大执行时间。
For Example, 如果要将最大执行时间设置为 120 秒,则设置值如下,
1 |
max_execution_time = 120 |
Q #12) PHP中的“通过值和引用传递变量”是什么意思??
Answer: 当变量作为值传递时,它被称为按值传递变量。
在这里,即使传递的变量发生变化,主变量也保持不变。
Sample code:
1 2 3 4 5 6 7 |
function test($n) { $n=$n+10; } $m=5; test($m); echo $m; |
当变量作为引用传递时,它被称为通过引用传递变量。这里,主变量和传递的变量共享相同的内存位置, & 用于引用。
因此,如果一个变量发生变化,那么另一个变量也会发生变化。
Sample code:
1 2 3 4 5 6 |
function test(&$n) { $n=$n+10; } $m=5; test($m); echo $m; |
Q #13) 解释类型转换和类型杂耍(type juggling).
Answer: PHP 可以为任何变量分配特定数据类型的方式称为类型转换。变量前面的括号中提到了所需的变量类型。
Sample code:
1 2 |
$str = "10"; // $str is now string $bool = (boolean) $str; // $bool is now boolean |
PHP 不支持变量声明的数据类型。变量的类型会根据分配的值自动更改,称为类型杂耍。
Sample code:
1 2 |
$val = 5; // $val is now number $val = "500" //$val is now string |
Q #14) 如何使用 PHP 与 MySQL 服务器建立连接?
Answer: 您必须提供 MySQL 主机名、用户名和密码才能在 mysqli_connect() 方法或声明 mysqli 类的数据库对象中与 MySQL 服务器建立连接。
Sample code:
1 2 |
$mysqli = mysqli_connect("localhost","username","password"); $mysqli = new mysqli("localhost","username","password"); |
Q #15) 如何使用 PHP 从 MySQL 数据库中检索数据?
Answer: PHP 中有许多函数可用于从 MySQL 数据库中检索数据.
下面提到的几个函数:
a) mysqli_fetch_array() – It is used to fetch the records as a numeric array or an associative array.
Sample code:
1 2 3 4 5 6 7 |
// Associative or Numeric array $result=mysqli_query($DBconnection,$query); $row=mysqli_fetch_array($result,MYSQLI_ASSOC); echo "Name is $row[0] "; echo "Email is $row['email'] "; |
b) mysqli_fetch_row() – It is used to fetch the records in a numeric array.
Sample code:
1 2 3 4 |
//Numeric array $result=mysqli_query($DBconnection,$query); $row=mysqli_fetch_array($result); printf ("%s %s\n",$row[0],$row[1]); |
c) mysqli_fetch_assoc() – It is used to fetch the records in an associative array.
Sample code:
1 2 3 4 |
// Associative array $result=mysqli_query($DBconnection,$query); $row=mysqli_fetch_array($result); printf ("%s %s\n",$row["name"],$row["email"]); |
d) mysqli_fetch_object() – It is used to fetch the records as an object.
Sample code:
1 2 3 4 |
// Object $result=mysqli_query($DBconnection,$query); $row=mysqli_fetch_array($result); printf ("%s %s\n",$row->name,$row->email); |
Q #16) mysqli_connect 和 mysqli_pconnect 有什么区别?
Answer:
mysqli_pconnect() 函数用于与脚本结束还和数据库建立持久连接。
mysqli_connect() 函数首先搜索任何现有的持久连接,如果不存在持久连接,那么它将创建一个新的数据库连接并在脚本末尾终止连接。.
Sample code:
1 2 3 4 5 6 |
$DBconnection = mysqli_connect("localhost","username","password","dbname"); // Check for valid connection if (mysqli_connect_errno()) { echo "Unable to connect with MySQL: " . mysqli_connect_error(); } |
mysqli_pconnect() function is depreciated in the new version of PHP, but you can create a persistence connection using mysqli_connect with the prefix p.
Q #17) PHP中使用哪个函数来计算任何查询返回的总行数?
Answer:
mysqli_num_rows() function 用于统计查询返回的总行数.
Sample code:
1 2 3 |
$mysqli = mysqli_connect("hostname","username","password","DBname"); $result=mysqli_query($mysqli,"select * from employees"); $count=mysqli_num_rows($result); |
Q #18) 如何在 PHP 中创建会话?
Answer:
session_start() function 用于在 PHP 中创建会话.
Sample code:
1 2 3 |
session_start(); //Start session $_SESSION['USERNAME']='Fahmida'; //Set a session value unset($_SESSION['USERNAME']; //delete session value |
Q #19) imagetypes() 方法有什么用?
Answer: image types() function r返回已安装 PHP 版本的支持图像列表。您可以使用此函数检查 PHP 是否支持特定的图像扩展。
Sample code:
1 2 3 4 |
//Check BMP extension is supported by PHP or not if (imagetypes() &IMG_BMP) { echo "BMP extension Support is enabled"; } |
Q #20) 您可以在 PHP 中使用哪个函数来打开文件以进行读取或写入或两者兼而有之?
Answer: You can use fopen() function 打开文件以进行读取或写入或两者兼而有之
.
Sample code:
1 2 3 |
$file1 = fopen("myfile1.txt","r"); //Open for reading $file2 = fopen("myfile2.txt","w"); //Open for writing $file3 = fopen("myfile3.txt","r+"); //Open for reading and writing |
Q #21) include() 和 require() 有什么区别?
Answer: include() 和 require() 函数都用于将 PHP 脚本从一个文件包含到另一个文件。但是这些功能之间是有区别的。
如果在使用 include() 函数包含文件时发生任何错误,则它会在显示错误消息后继续执行脚本。 require() 函数通过在发生错误时显示错误消息来停止脚本的执行。
Sample code:
1 2 |
if (!include(‘test.php’)) echo “Error in file inclusion”; if (!require(‘test.php’)) echo “Error in file inclusion”; |
Q #22) PHP中使用哪个函数删除文件?
Answer:
unlink() function is used in PHP to 删除文件。
Sample code:
1 |
unlink('filename'); |
Q #23) strip_tags() 方法有什么用?
Answer: strip_tags() function 用于通过省略 HTML、XML 和 PHP 标记从文本中检索字符串。该函数有一个强制参数和一个可选参数。可选参数用于接受特定标签。
Sample code:
1 2 3 4 |
//Remove all tags from the text echo strip_tags("<b>PHP</b> is a popular <em>scripting</em> language"); //Remove all tags excluding <b> tag echo strip_tags("<b>PHP</b> is a popular <em>scripting</em> language","<b>"); |
Q #24) 如何在 PHP 中向客户端发送 HTTP 标头?
Answer: header() 函数用于在发送任何输出之前将原始 HTTP 标头发送到客户端。
Sample code:
1 |
header('Location: http://www.your_domain/'); |
Q #25) PHP中有哪些函数用于统计数组元素的总数?
Answer: count() 和 sizeof() 函数可用于计算 PHP 中数组元素的总数.
Sample code:
1 2 3 4 |
$names=array(“Asa”,”Prinka”,”Abhijeet”); echo count($names); $marks=array(95,70,87); echo sizeof($marks); |
Q #26) substr() 和 strstr() 有什么区别?
Answer:
substr() 函数根据起点和长度返回字符串的一部分。此函数的长度参数是可选的,如果省略它,则将返回从起点开始的字符串的剩余部分。
strstr() 函数在另一个字符串中搜索第一次出现的字符串。此函数的第三个参数是可选的,它用于检索在搜索字符串第一次出现之前出现的字符串部分。
Sample code:
1 2 |
echo substr("Computer Programming",9,7); //Returns “Program” echo substr("Computer Programming",9); //Returns “Programming” |
Sample code:
1 2 |
echo strstr("Learning Laravel 5!","Laravel"); //Returns Laravel 5! echo strstr("Learning Laravel 5!","Laravel",true); //Returns Learning |
Q #27) 如何使用 PHP 上传文件?
Answer: 要使用 PHP 上传文件,您必须执行以下任务:
(i) 启用 file_uploads 指令
Open php.ini file and find out the file_uploads directive and make it on.
1 |
file_uploads = On |
(ii) 使用 enctype 属性和文件元素创建 HTML 表单以上传文件。
1 2 3 4 |
<form action="upload.php" method="post" enctype="multipart/form-data"> <input type="file" name="upd" id="upd"> <input type="submit" value="Upload" name="upload"> </form> |
(iii) 编写一个PHP脚本来上传文件
1 2 3 4 5 |
if (move_uploaded_file($_FILES["upd"]["tmp_name"], "Uploads/")) { echo "The file ". basename( $_FILES["upd"]["name"]). " is uploaded."; } else { echo "There is an error in uploading."; } |
Q #28) 如何在 PHP 中声明一个常量变量?
Answer: define() 函数用于在 PHP 中声明一个常量变量。常量变量声明不带 $ 符号。
Sample code:
1 |
define("PI",3.14); |
Q #29) PHP中使用哪个函数来搜索数组中的特定值?
Answer: in_array() 函数用于搜索数组中的特定值.
Sample code:
1 2 3 4 5 6 7 |
$languages = array("C#", "Java", "PHP", "VB.Net"); if (in_array("PHP", $languages)) { echo "PHP is in the list"; } else { echo "php is not in the list"; } |
Q #30) $_REQUEST 变量的用途是什么?
Answer: $_REQUEST 变量用于从提交的 HTML 表单中读取数据。
Sample code:
Here, the $_REQUEST variable is used to read the submitted form field with the name ‘username’. If the form is submitted without any value, then it will print as “Name is empty”, otherwise it will print the submitted value.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
<?php if (isset($_POST['submit'])) { // collect value of input field $name = $_REQUEST['username']; if (empty($name)) { echo "Name is empty"; } else { echo $name; } } else { ?> <form method="post" action="#"> Name: <input type="text" name="username"> <input type="submit" name="submit"> </form> <?php } ?> |
Q #31) PHP中for和foreach循环有什么区别?
Answer: For循环主要用于迭代预先定义的次数,Foreach循环用于读取数组元素或MySQL结果集,其中迭代次数可能未知。
Sample code:
1 2 3 4 |
//Loop will iterate for 5 times for ($n = 0; $n <= 5; $n++) { echo "The number is: $n <br>"; } |
Sample code:
1 2 3 4 |
//Loop will iterate based on array elements $parts = array("HDD", "Monitor", "Mouse", "Keyboard"); foreach ($parts as $value) { echo "$value <br>"; } |
Q #32) PHP会话持续多长时间?
Answer: 默认情况下,会话数据在 PHP 中将持续 24 分钟或 1440 秒。但是,如果您愿意,可以通过修改 php.ini 文件中 gc_maxlifetime 指令的值来更改持续时间。要将会话时间设置为 30 分钟,请打开 php.ini 文件并设置 gc_maxlifetime 指令的值,如下所示,
gc_maxlifetime = 1800
Q #33) “= =”和“= = =”运算符有什么区别?
Answer: “= = =”被称为严格等价运算符,用于通过比较数据类型和值来检查两个值的等价性。
Sample code:
10 and “10” are equal by values but are not equal by data type. One is a string and one is a number. So, if the condition will be false and print “n is not equal to 10”.
1 2 3 4 5 |
$n = 10; if ($n === "10") echo "n is equal to 10"; else echo "n is not equal to 10"; //This will print |
Q #34) PHP中哪个运算符用于组合字符串值?
Answer: 可以使用“.”运算符组合两个或多个字符串值。
Sample code:
1 2 3 |
$val1 = "Software "; $val2 = "Testing"; echo $val1.$val2; // The output is “Software Testing” |
Q #35) 什么是 PEAR?
Answer: PEAR 的完整形式是“PHP Extension and Application Repository”。
任何人都可以通过使用这个框架免费下载可重用的 PHP 组件。它包含来自不同开发人员的不同类型的包。
Q #36) PHP中可能会发生哪些类型的错误?
Answer:PHP中可能出现不同类型的错误.
Some major error types are mentioned below:
- Fatal Errors– The execution of the script stops when this error occurs.
Sample code:
In the following script, f1() function is declared but f2() function is called which is not declared. The execution of the script will stop when f2() function will call. So, “Testing Fatal Error” will not be printed.
1 2 3 4 5 |
function f1() { echo "function 1"; } f2(); echo “Testing Fatal Error”; |
- Parse Errors– This type of error occurs when the coder uses a wrong syntax in the script.
Sample code:
Here, semicolon(;) is missing at the end of the first echo statement.
1 2 |
echo "This is a testing script&lt;br/&gt;" echo "error"; |
- Warning Errors- This type of error does not stop the execution of a script. It continues the script even after displaying the error.
Sample code:
In the following script, if the test.txt file does not exist in the current location then a warning message will display to show the error and print “Opening File” text by continuing the execution.
1 2 |
$handler = fopen("test.txt","r"); echo "Opening File"; |
- Notice Errors- This type of error shows a minor error of the script and continues the execution after displaying the error.
Here, the variable, $a is defined but $b is not defined. So, a notice of the undefined variable will display for “echo $b” statement and print “Checking notice error” by continuing the script.
Sample code:
1 2 3 |
$a = 100; echo $b; echo "Checking notice error"; |
Q #37) PHP是否支持多重继承?
Answer: PHP 不支持多重继承。为了实现多继承的特性,在 PHP 中使用了接口。
Sample code:
这里在一个类中声明并实现了两个接口 Isbn 和 Type,详细介绍 PHP 中添加多继承的特性。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
interface Isbn { public function setISBN($isbn); } interface Type{ public function setType($type); } class bookDetails implements Isbn, Type { private $isbn; private $type; public function setISBN($isbn) { $this -> isbn = $isbn; } public function setType($type) { $this -> type = $type; } } |
Q #38) session和cookie有什么区别?
答:会话是一个全局变量,在服务器中用于存储会话数据。当新会话创建带有会话 ID 的 cookie 时,该 cookie 将存储在访问者的计算机上。 session 变量可以存储比 cookie 变量更多的数据。
会话数据存储在 $_SESSION 数组中,Cookie 数据存储在 $_COOKIE 数组中。访问者关闭浏览器时会自动删除会话值,并且不会自动删除 cookie 值。
Q #39) mysqli_real_escape_string()函数有什么用?
Answer: mysqli_real_escape_string() function is 用于从字符串中转义特殊字符以使用 SQL 语句。
Sample code:
1 2 3 |
$DBconnection=mysqli_connect("localhost","username","password","dbname"); $productName = mysqli_real_escape_string($con, $_POST['proname']); $ProductType = mysqli_real_escape_string($con, $_POST['protype']); |
Q #40) 哪些函数用于从字符串中删除空格?
Answer: PHP中有三个函数可以从字符串中删除空格.
- trim() – It removes whitespaces from the left and right side of the string.
- ltrim() – It removes whitespaces from the left side of the string.
- rtrim() – It removes whitespaces from the right side of the string.
Sample code:
1 2 3 4 |
$str = " Tutorials for your help"; $val1 = trim($str); $val2 = ltrim($str); $val3 = rtrim($str); |
Q #41) 什么是持久性 cookie?
Answer: 永久存储在浏览器中的 cookie 文件称为持久性 cookie。它不安全,主要用于长时间跟踪访客。
This type of cookie can be declared as follows,
1 |
setccookie ("cookie_name", "cookie_value", strtotime("+2 years"); |
Q #42) PHP如何防止跨站脚本攻击?
Answer: PHP的htmlentities()函数可用于防止跨站脚本攻击.
Q #43) 哪个 PHP 全局变量用于上传文件?
Answer: $_FILE[] 数组包含上传文件的所有信息.
The use of various indexes of this array is mentioned below:
- $_FILES[$fieldName][‘name’] – Keeps the original file name.
- $_FILES[$fieldName][‘type’] – Keeps the file type of an uploaded file.
- $_FILES[$fieldName][‘size’] – Stores the file size in bytes.
- $_FILES[$fieldName][‘tmp_name’] – Keeps the temporary file name which is used to store the file in the server.
- $_FILES[$fieldName][‘error’] – Contains error code related to the error that appears during the upload.
Q #44) 公共、私有、受保护、静态和final范围的含义?
Answer:
- public——声明为公共的变量、类和方法可以从任何地方访问。
- private——声明为私有的变量、类和方法只能由父类访问。
- protected ——声明为受保护的变量、类和方法只能由父类和子类访问。
- static ——声明为静态的变量可以在失去作用域后保持该值。
- final——这个范围防止子类再次声明同一个项目。
final
是在 PHP5 版本引入的,它修饰的类不允许被继承,它修饰的方法不允许被重写。
Q #45) 如何在 PHP 中检索图像属性?
Answer:
- getimagesize() – It is used to get the image size. (图像大小)
- exif_imagetype() – It is used to get the image type. (图像类型)
- imagesx() – It is used to get the image width. (图像宽)
- imagesy() – It is used to get the image height.(图像高)
Q #46) 抽象类和接口有什么区别?
Answer:
- Abstract classes are used for closely related objects and interfaces are used for unrelated objects.
- PHP class can implement multiple interfaces but can’t inherit multiple abstract classes.
- Common behavior can be implemented in the abstract class but not an interface.
Q #47) 什么是垃圾回收?
Answer: It is an automated feature of PHP.
When it runs, it removes all session data which are not accessed for a long time. It runs on /tmp directory which is the default session directory.
PHP directives which are used for garbage collection include:
- session.gc_maxlifetime (default value, 1440)
- session.gc_probability (default value, 1)
- session.gc_divisor (default value, 100)
Q #48) PHP中使用哪个库来做各种类型的Image工作?
Answer: Using the GD library, various types of image work can be done in PHP. Image work includes rotating images, cropping an image, creating image thumbnail, etc.
Q #49) 什么是 URL 重写?
Answer: Appending the session ID in every local URL of the requested page for keeping the session information is called URL rewriting.
The disadvantages of these methods are, it doesn’t allow persistence between the sessions and, the user can easily copy and paste the URL and send it to another user.
Q #50) 什么是 PDO?
Answer: PDO 代表 PHP Data Objects.
It is a lightweight PHP extension that uses a consistence interface for accessing the database. Using PDO, a developer can easily switch from one database server to the other. But it does not support all the advanced features of the new MySQL server.
Conclusion
I hope, this article will increase your confidence level to face any PHP interview. Feel free to contact us and suggest missing PHP Interview questions that you face in an interview.
Wish you all success for your interview!!