在本教程中,您将学习有关 Python 集的所有知识。 如何创建它们,从中添加元素或从中删除元素,以及在 Python 中对集合执行的所有操作。
集合是项目的无序集合。 每个集的元素都是唯一的(没有重复),并且必须是不可变的(无法更改)。
但是,集合本身是可变的。 我们可以从中添加或删除项目。
集还可以用于执行数学集运算,例如并集,交集,对称差等。
创建 Python 集
通过将所有项目(元素)放置在大括号{}
中(用逗号分隔)或使用内置的set()
函数,可以创建一个集合。
它可以具有任意数量的项目,并且它们可以具有不同的类型(整数,浮点数,元组,字符串等)。 但是集合不能具有可变元素,例如列表,集合或字典作为其元素。
1 2 3 4 5 6 7 8 |
<span class="pl-c"># Different types of sets in Python</span> <span class="pl-c"># set of integers</span> <span class="pl-s1">my_set</span> <span class="pl-c1">=</span> {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>} <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) <span class="pl-c"># set of mixed datatypes</span> <span class="pl-s1">my_set</span> <span class="pl-c1">=</span> {<span class="pl-c1">1.0</span>, <span class="pl-s">"Hello"</span>, (<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>)} <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) |
输出
1 2 |
{<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>} {<span class="pl-c1">1.0</span>, (<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>), <span class="pl-s">'Hello'</span>} |
也尝试以下示例。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
<span class="pl-c"># set cannot have duplicates</span> <span class="pl-c"># Output: {1, 2, 3, 4}</span> <span class="pl-s1">my_set</span> <span class="pl-c1">=</span> {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">3</span>, <span class="pl-c1">2</span>} <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) <span class="pl-c"># we can make set from a list</span> <span class="pl-c"># Output: {1, 2, 3}</span> <span class="pl-s1">my_set</span> <span class="pl-c1">=</span> <span class="pl-en">set</span>([<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">2</span>]) <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) <span class="pl-c"># set cannot have mutable items</span> <span class="pl-c"># here [3, 4] is a mutable list</span> <span class="pl-c"># this will cause an error.</span> <span class="pl-s1">my_set</span> <span class="pl-c1">=</span> {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, [<span class="pl-c1">3</span>, <span class="pl-c1">4</span>]} |
输出:
1 2 3 4 5 6 |
{<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>} {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>} <span class="pl-v">Traceback</span> (<span class="pl-s1">most</span> <span class="pl-s1">recent</span> <span class="pl-s1">call</span> <span class="pl-s1">last</span>): <span class="pl-v">File</span> <span class="pl-s">"<string>"</span>, <span class="pl-s1">line</span> <span class="pl-c1">15</span>, <span class="pl-s1">in</span> <span class="pl-c1"><</span><span class="pl-s1">module</span><span class="pl-c1">></span> <span class="pl-s1">my_set</span> <span class="pl-c1">=</span> {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, [<span class="pl-c1">3</span>, <span class="pl-c1">4</span>]} <span class="pl-v">TypeError</span>: <span class="pl-s1">unhashable</span> <span class="pl-s1">type</span>: <span class="pl-s">'list'</span> |
创建一个空集有点棘手。
空花括号{}
将在 Python 中创建一个空字典。 为了创建没有任何元素的集合,我们使用不带任何参数的set()
函数。
1 2 3 4 5 6 7 8 9 10 11 12 13 |
<span class="pl-c"># Distinguish set and dictionary while creating empty set</span> <span class="pl-c"># initialize a with {}</span> <span class="pl-s1">a</span> <span class="pl-c1">=</span> {} <span class="pl-c"># check data type of a</span> <span class="pl-en">print</span>(<span class="pl-en">type</span>(<span class="pl-s1">a</span>)) <span class="pl-c"># initialize a with set()</span> <span class="pl-s1">a</span> <span class="pl-c1">=</span> <span class="pl-en">set</span>() <span class="pl-c"># check data type of a</span> <span class="pl-en">print</span>(<span class="pl-en">type</span>(<span class="pl-s1">a</span>)) |
输出:
1 2 |
<span class="pl-c1"><</span><span class="pl-k">class</span> <span class="pl-s">'dict'</span><span class="pl-c1">></span> <span class="pl-c1"><</span><span class="pl-k">class</span> <span class="pl-s">'set'</span><span class="pl-c1">></span> |
在 Python 中修改集
集是可变的。 但是,由于它们是无序的,因此索引没有意义。
我们无法使用索引或切片来访问或更改集合的元素。 设置数据类型不支持它。
我们可以使用add()
方法添加一个元素,并使用update()
方法添加多个元素。update()
方法可以将元组,列表,字符串或其他集合用作其参数。 在所有情况下,都避免重复。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
<span class="pl-c"># initialize my_set</span> <span class="pl-s1">my_set</span> <span class="pl-c1">=</span> {<span class="pl-c1">1</span>, <span class="pl-c1">3</span>} <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) <span class="pl-c"># if you uncomment line 9,</span> <span class="pl-c"># you will get an error</span> <span class="pl-c"># TypeError: 'set' object does not support indexing</span> <span class="pl-c"># my_set[0]</span> <span class="pl-c"># add an element</span> <span class="pl-c"># Output: {1, 2, 3}</span> <span class="pl-s1">my_set</span>.<span class="pl-en">add</span>(<span class="pl-c1">2</span>) <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) <span class="pl-c"># add multiple elements</span> <span class="pl-c"># Output: {1, 2, 3, 4}</span> <span class="pl-s1">my_set</span>.<span class="pl-en">update</span>([<span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>]) <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) <span class="pl-c"># add list and set</span> <span class="pl-c"># Output: {1, 2, 3, 4, 5, 6, 8}</span> <span class="pl-s1">my_set</span>.<span class="pl-en">update</span>([<span class="pl-c1">4</span>, <span class="pl-c1">5</span>], {<span class="pl-c1">1</span>, <span class="pl-c1">6</span>, <span class="pl-c1">8</span>}) <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) |
输出:
1 2 3 4 |
{<span class="pl-c1">1</span>, <span class="pl-c1">3</span>} {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>} {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>} {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>, <span class="pl-c1">8</span>} |
从集中删除元素
可以使用discard()
和remove()
方法从集合中移除特定项目。
两者之间的唯一区别是,如果元素中不存在discard()
函数,则该集合将保持不变。 另一方面,在这种情况下,remove()
函数将引发错误(如果集合中不存在元素)。
以下示例将说明这一点。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
<span class="pl-c"># Difference between discard() and remove()</span> <span class="pl-c"># initialize my_set</span> <span class="pl-s1">my_set</span> <span class="pl-c1">=</span> {<span class="pl-c1">1</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>} <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) <span class="pl-c"># discard an element</span> <span class="pl-c"># Output: {1, 3, 5, 6}</span> <span class="pl-s1">my_set</span>.<span class="pl-en">discard</span>(<span class="pl-c1">4</span>) <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) <span class="pl-c"># remove an element</span> <span class="pl-c"># Output: {1, 3, 5}</span> <span class="pl-s1">my_set</span>.<span class="pl-en">remove</span>(<span class="pl-c1">6</span>) <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) <span class="pl-c"># discard an element</span> <span class="pl-c"># not present in my_set</span> <span class="pl-c"># Output: {1, 3, 5}</span> <span class="pl-s1">my_set</span>.<span class="pl-en">discard</span>(<span class="pl-c1">2</span>) <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) <span class="pl-c"># remove an element</span> <span class="pl-c"># not present in my_set</span> <span class="pl-c"># you will get an error.</span> <span class="pl-c"># Output: KeyError</span> <span class="pl-s1">my_set</span>.<span class="pl-en">remove</span>(<span class="pl-c1">2</span>) |
输出:
1 2 3 4 5 6 7 |
{<span class="pl-c1">1</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>} {<span class="pl-c1">1</span>, <span class="pl-c1">3</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>} {<span class="pl-c1">1</span>, <span class="pl-c1">3</span>, <span class="pl-c1">5</span>} {<span class="pl-c1">1</span>, <span class="pl-c1">3</span>, <span class="pl-c1">5</span>} <span class="pl-v">Traceback</span> (<span class="pl-s1">most</span> <span class="pl-s1">recent</span> <span class="pl-s1">call</span> <span class="pl-s1">last</span>): <span class="pl-v">File</span> <span class="pl-s">"<string>"</span>, <span class="pl-s1">line</span> <span class="pl-c1">28</span>, <span class="pl-s1">in</span> <span class="pl-c1"><</span><span class="pl-s1">module</span><span class="pl-c1">></span> <span class="pl-v">KeyError</span>: <span class="pl-c1">2</span> |
同样,我们可以使用pop()
方法删除并返回一个项目。
由于集是无序数据类型,因此无法确定将弹出哪个项目。 这是完全任意的。
我们还可以使用clear()
方法从集合中删除所有项目。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
<span class="pl-c"># initialize my_set</span> <span class="pl-c"># Output: set of unique elements</span> <span class="pl-s1">my_set</span> <span class="pl-c1">=</span> <span class="pl-en">set</span>(<span class="pl-s">"HelloWorld"</span>) <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) <span class="pl-c"># pop an element</span> <span class="pl-c"># Output: random element</span> <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>.<span class="pl-en">pop</span>()) <span class="pl-c"># pop another element</span> <span class="pl-s1">my_set</span>.<span class="pl-en">pop</span>() <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) <span class="pl-c"># clear my_set</span> <span class="pl-c"># Output: set()</span> <span class="pl-s1">my_set</span>.<span class="pl-en">clear</span>() <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) <span class="pl-en">print</span>(<span class="pl-s1">my_set</span>) |
输出:
1 2 3 4 |
{<span class="pl-s">'H'</span>, <span class="pl-s">'l'</span>, <span class="pl-s">'r'</span>, <span class="pl-s">'W'</span>, <span class="pl-s">'o'</span>, <span class="pl-s">'d'</span>, <span class="pl-s">'e'</span>} <span class="pl-v">H</span> {<span class="pl-s">'r'</span>, <span class="pl-s">'W'</span>, <span class="pl-s">'o'</span>, <span class="pl-s">'d'</span>, <span class="pl-s">'e'</span>} <span class="pl-en">set</span>() |
Python 集操作
集合可用于执行数学集合运算,例如并集,交集,差和对称差。 我们可以使用运算符或方法来做到这一点。
让我们考虑以下两组用于以下操作。
1 2 |
<span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">A</span> <span class="pl-c1">=</span> {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">5</span>} <span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">B</span> <span class="pl-c1">=</span> {<span class="pl-c1">4</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>, <span class="pl-c1">7</span>, <span class="pl-c1">8</span>} |
并集
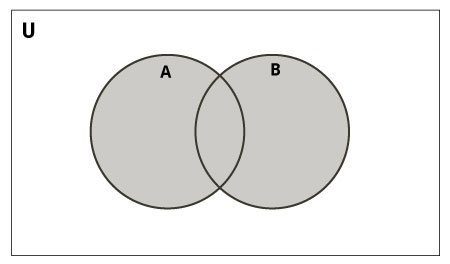
Python 中的并集
A
和B
的并集是两个集合中所有元素的集合。
联合使用|
运算符执行。 使用union()
方法可以完成相同的操作。
1 2 3 4 5 6 7 8 |
<span class="pl-c"># Set union method</span> <span class="pl-c"># initialize A and B</span> <span class="pl-v">A</span> <span class="pl-c1">=</span> {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">5</span>} <span class="pl-v">B</span> <span class="pl-c1">=</span> {<span class="pl-c1">4</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>, <span class="pl-c1">7</span>, <span class="pl-c1">8</span>} <span class="pl-c"># use | operator</span> <span class="pl-c"># Output: {1, 2, 3, 4, 5, 6, 7, 8}</span> <span class="pl-en">print</span>(<span class="pl-v">A</span> <span class="pl-c1">|</span> <span class="pl-v">B</span>) |
输出:
1 |
{<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>, <span class="pl-c1">7</span>, <span class="pl-c1">8</span>} |
在 Python shell 上尝试以下示例。
1 2 3 4 5 6 7 |
<span class="pl-c"># use union function</span> <span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">A</span>.<span class="pl-en">union</span>(<span class="pl-v">B</span>) {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>, <span class="pl-c1">7</span>, <span class="pl-c1">8</span>} <span class="pl-c"># use union function on B</span> <span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">B</span>.<span class="pl-en">union</span>(<span class="pl-v">A</span>) {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>, <span class="pl-c1">7</span>, <span class="pl-c1">8</span>} |
交集
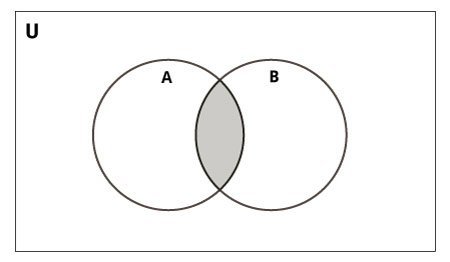
Python 中的交集
A
和B
的交集是在这两组中都相同的一组元素。
交叉使用&
运算符执行。 使用intersection()
方法可以完成相同的操作。
1 2 3 4 5 6 7 8 |
<span class="pl-c"># Intersection of sets</span> <span class="pl-c"># initialize A and B</span> <span class="pl-v">A</span> <span class="pl-c1">=</span> {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">5</span>} <span class="pl-v">B</span> <span class="pl-c1">=</span> {<span class="pl-c1">4</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>, <span class="pl-c1">7</span>, <span class="pl-c1">8</span>} <span class="pl-c"># use & operator</span> <span class="pl-c"># Output: {4, 5}</span> <span class="pl-en">print</span>(<span class="pl-v">A</span> <span class="pl-c1">&</span> <span class="pl-v">B</span>) |
输出:
1 |
{<span class="pl-c1">4</span>, <span class="pl-c1">5</span>} |
在 Python Shell 中尝试以下程序:
1 2 3 4 5 6 7 |
<span class="pl-c"># use intersection function on A</span> <span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">A</span>.<span class="pl-en">intersection</span>(<span class="pl-v">B</span>) {<span class="pl-c1">4</span>, <span class="pl-c1">5</span>} <span class="pl-c"># use intersection function on B</span> <span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">B</span>.<span class="pl-en">intersection</span>(<span class="pl-v">A</span>) {<span class="pl-c1">4</span>, <span class="pl-c1">5</span>} |
差集
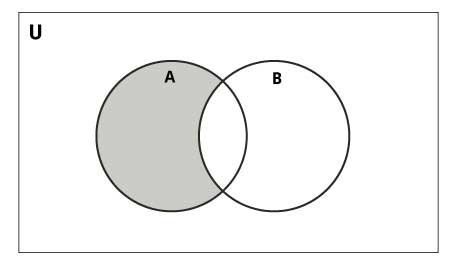
Python 中的差集
集合B
与集合A
(A
– B
)的区别是仅在A
中的一组元素,但不在B
中。 类似地,B
– A
是B
中的一组元素,但不是A
中的一组元素。
使用-
运算符进行区别。 使用difference()
方法可以完成相同的操作。
1 2 3 4 5 6 7 8 |
<span class="pl-c"># Difference of two sets</span> <span class="pl-c"># initialize A and B</span> <span class="pl-v">A</span> <span class="pl-c1">=</span> {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">5</span>} <span class="pl-v">B</span> <span class="pl-c1">=</span> {<span class="pl-c1">4</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>, <span class="pl-c1">7</span>, <span class="pl-c1">8</span>} <span class="pl-c"># use - operator on A</span> <span class="pl-c"># Output: {1, 2, 3}</span> <span class="pl-en">print</span>(<span class="pl-v">A</span> <span class="pl-c1">-</span> <span class="pl-v">B</span>) |
输出:
1 |
{<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>} |
在 Python Shell 中尝试以下程序:
1 2 3 4 5 6 7 8 9 10 11 |
<span class="pl-c"># use difference function on A</span> <span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">A</span>.<span class="pl-en">difference</span>(<span class="pl-v">B</span>) {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>} <span class="pl-c"># use - operator on B</span> <span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">B</span> <span class="pl-c1">-</span> <span class="pl-v">A</span> {<span class="pl-c1">8</span>, <span class="pl-c1">6</span>, <span class="pl-c1">7</span>} <span class="pl-c"># use difference function on B</span> <span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">B</span>.<span class="pl-en">difference</span>(<span class="pl-v">A</span>) {<span class="pl-c1">8</span>, <span class="pl-c1">6</span>, <span class="pl-c1">7</span>} |
对称差集
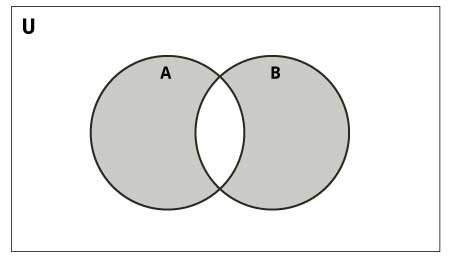
Python 的对称差集
A
和B
的对称差异是A
和B
中的一组元素,但两者都不相同(不包括交叉点)。
对称差使用^
运算符执行。 使用方法symmetric_difference()
可以完成相同的操作。
1 2 3 4 5 6 7 8 |
<span class="pl-c"># Symmetric difference of two sets</span> <span class="pl-c"># initialize A and B</span> <span class="pl-v">A</span> <span class="pl-c1">=</span> {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">5</span>} <span class="pl-v">B</span> <span class="pl-c1">=</span> {<span class="pl-c1">4</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>, <span class="pl-c1">7</span>, <span class="pl-c1">8</span>} <span class="pl-c"># use ^ operator</span> <span class="pl-c"># Output: {1, 2, 3, 6, 7, 8}</span> <span class="pl-en">print</span>(<span class="pl-v">A</span> <span class="pl-c1">^</span> <span class="pl-v">B</span>) |
输出:
1 |
{<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">6</span>, <span class="pl-c1">7</span>, <span class="pl-c1">8</span>} |
在 Python Shell 中尝试以下程序:
1 2 3 4 5 6 7 |
<span class="pl-c"># use symmetric_difference function on A</span> <span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">A</span>.<span class="pl-en">symmetric_difference</span>(<span class="pl-v">B</span>) {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">6</span>, <span class="pl-c1">7</span>, <span class="pl-c1">8</span>} <span class="pl-c"># use symmetric_difference function on B</span> <span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">B</span>.<span class="pl-en">symmetric_difference</span>(<span class="pl-v">A</span>) {<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">6</span>, <span class="pl-c1">7</span>, <span class="pl-c1">8</span>} |
其他 Python 集方法
设置方法有很多,上面已经使用了其中的一些方法。 这是设置对象可用的所有方法的列表:
方法 | 描述 |
---|---|
add() |
将元素添加到集合中 |
clear() |
从集合中删除所有元素 |
copy() |
返回集合的副本 |
difference() |
将两个或多个集合的差返回为新集合 |
difference_update() |
从该集合中删除另一个集合的所有元素 |
reject() |
如果元素是成员,则从集合中删除它。 (如果元素不在集合中,则不执行任何操作) |
intersection() |
返回两个集合的交集作为新集合 |
intersection_update() |
用自身和另一个的交集更新集合 |
isdisjoint() |
如果两个集合的交点为空,则返回True |
issubset() |
如果另一个集合包含此集合,则返回True |
issuperset() |
如果此集合包含另一个集合,则返回True |
pop() |
删除并返回一个任意集的元素。 如果集合为空,则升起KeyError |
remove() |
从集合中删除一个元素。 如果元素不是成员,则引发KeyError |
symmetric_difference() |
将两个集合的对称差作为新集合返回 |
symmetric_difference_update() |
用本身和另一个的对称差异更新一个集合 |
union() |
返回新集合中集合的并集 |
update() |
用自身和他人的并集更新集合 |
其他集操作
集的成员资格测试
我们可以使用in
关键字来测试项目是否存在于集合中。
1 2 3 4 5 6 7 8 9 10 11 |
<span class="pl-c"># in keyword in a set</span> <span class="pl-c"># initialize my_set</span> <span class="pl-s1">my_set</span> <span class="pl-c1">=</span> <span class="pl-en">set</span>(<span class="pl-s">"apple"</span>) <span class="pl-c"># check if 'a' is present</span> <span class="pl-c"># Output: True</span> <span class="pl-en">print</span>(<span class="pl-s">'a'</span> <span class="pl-c1">in</span> <span class="pl-s1">my_set</span>) <span class="pl-c"># check if 'p' is present</span> <span class="pl-c"># Output: False</span> <span class="pl-en">print</span>(<span class="pl-s">'p'</span> <span class="pl-c1">not</span> <span class="pl-c1">in</span> <span class="pl-s1">my_set</span>) |
输出:
1 2 |
<span class="pl-c1">True</span> <span class="pl-c1">False</span> |
遍历集
我们可以使用for
循环遍历集合中的每个项目。
1 2 3 4 5 6 7 |
<span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-k">for</span> <span class="pl-s1">letter</span> <span class="pl-c1">in</span> <span class="pl-en">set</span>(<span class="pl-s">"apple"</span>): ... <span class="pl-s1">print</span>(<span class="pl-s1">letter</span>) ... <span class="pl-s1">a</span> <span class="pl-s1">p</span> <span class="pl-s1">e</span> <span class="pl-s1">l</span> |
集的内置函数
诸如all()
,any()
,enumerate()
,len()
,max()
,min()
,sorted()
,sum()
等内置函数通常与集合一起使用以执行不同的任务。
| 函数 | Description |
| all()
| 如果集合的所有元素都为true
(或者集合为空),则返回True
。 |
| any()
| 如果集合中的任何元素为true
,则返回True
。 如果集合为空,则返回False
。 |
| enumerate()
| 返回一个枚举对象。 它包含该集合中所有项目的索引和值对。 |
| len()
| 返回集合中的长度(项目数)。 |
| max()
| 返回集合中最大的项目。 |
| min()
| 返回集合中最小的项目。 |
| sorted()
| 从集合中的元素返回一个新的排序列表(不对集合本身进行排序)。 |
| sum()
| 返回集合中所有元素的总和。 |
Python Frozenset
Frozenset
是具有集合特征的新类,但是一旦分配,就不能更改其元素。 元组是不可变列表,而冻结集是不可变集。
可变的集合不可散列,因此不能用作字典键。 另一方面,frozenset
是可哈希化的,可用作字典的键。
可以使用Frozenset()
函数创建Frozenset
。
此数据类型支持copy()
,difference()
,intersection()
,isdisjoint()
,issubset()
,issuperset()
,symmetric_difference()
和union()
之类的方法。 由于是不可变的,因此没有添加或删除元素的方法。
1 2 3 4 |
<span class="pl-c"># Frozensets</span> <span class="pl-c"># initialize A and B</span> <span class="pl-v">A</span> <span class="pl-c1">=</span> <span class="pl-en">frozenset</span>([<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>]) <span class="pl-v">B</span> <span class="pl-c1">=</span> <span class="pl-en">frozenset</span>([<span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>]) |
在 Python shell 上尝试这些示例。
1 2 3 4 5 6 7 8 9 |
<span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">A</span>.<span class="pl-en">isdisjoint</span>(<span class="pl-v">B</span>) <span class="pl-c1">False</span> <span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">A</span>.<span class="pl-en">difference</span>(<span class="pl-v">B</span>) <span class="pl-en">frozenset</span>({<span class="pl-c1">1</span>, <span class="pl-c1">2</span>}) <span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">A</span> <span class="pl-c1">|</span> <span class="pl-v">B</span> <span class="pl-en">frozenset</span>({<span class="pl-c1">1</span>, <span class="pl-c1">2</span>, <span class="pl-c1">3</span>, <span class="pl-c1">4</span>, <span class="pl-c1">5</span>, <span class="pl-c1">6</span>}) <span class="pl-c1">>></span><span class="pl-c1">></span> <span class="pl-v">A</span>.<span class="pl-en">add</span>(<span class="pl-c1">3</span>) ... <span class="pl-v">AttributeError</span>: <span class="pl-s">'frozenset'</span> <span class="pl-s1">object</span> <span class="pl-s1">has</span> <span class="pl-s1">no</span> <span class="pl-s1">attribute</span> <span class="pl-s">'add'</span> |